Android App development with I-SYST's BLE kits
It is easy to develop Android App using our BLE products
1. Required components
The following are needed for a full development environment:
- Android Studio
- Android App example with I-SYST BlUEPYRO-M3225
- Android App example with I-SYST BLUEIO-TAG-EVIM
- Android device (phone, tablet)
- I-SYST BLE devices
- BLUEPYRO-M3225
- BLUEIO-TAG-EVIM
2. Installing Android Studio
1. Start by downloading Android Studio here:
Download and install Android Studio to develop Android apps at the following link: Android Studio
Follow the link below to install Android Studio: Android Studio Install
2. Create new virtual device
After finishing installing Android Studio, create a new Android virtual device in AVD Manager
3. Import BluePyro Android Project
Download source code Android app at BluePyro Android Project
Import Android project BluePyro
Run the Bluepyro-M3225 Android App Project with a new Virtual device
Now it is ready to run on your device
Connect your Android device and run the project on your device. Test the Bluepyro-M3225 device with the Bluepyro app on your device. Now you are free to develop any android apps with Bluepyro-M3225.
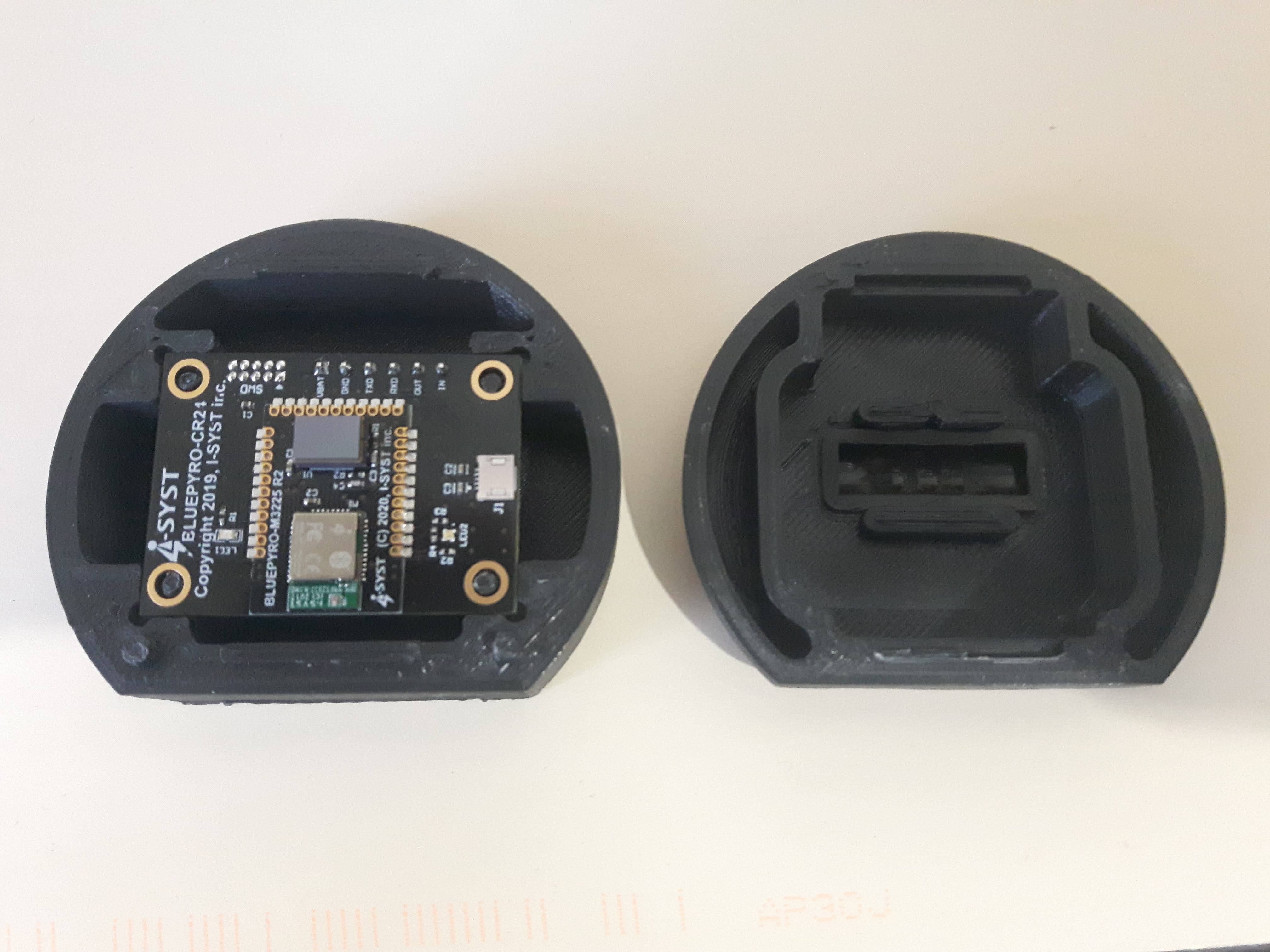
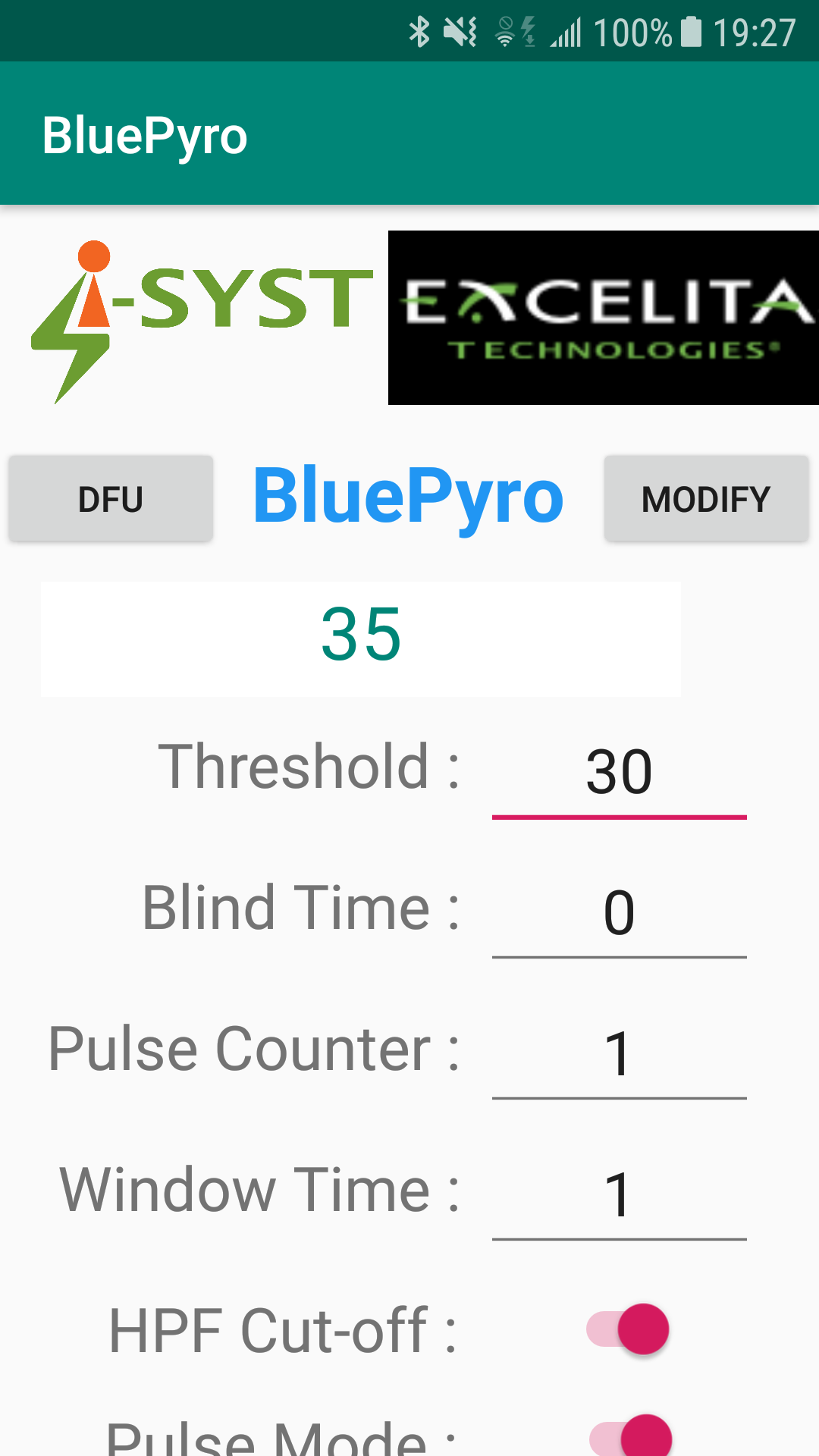
4. Import SensorTagDemo Android Project
Download source code Android app at SensorTagDemo Android Project
Import SensorTagDemo project
Connect your Android device and run the project on your device.
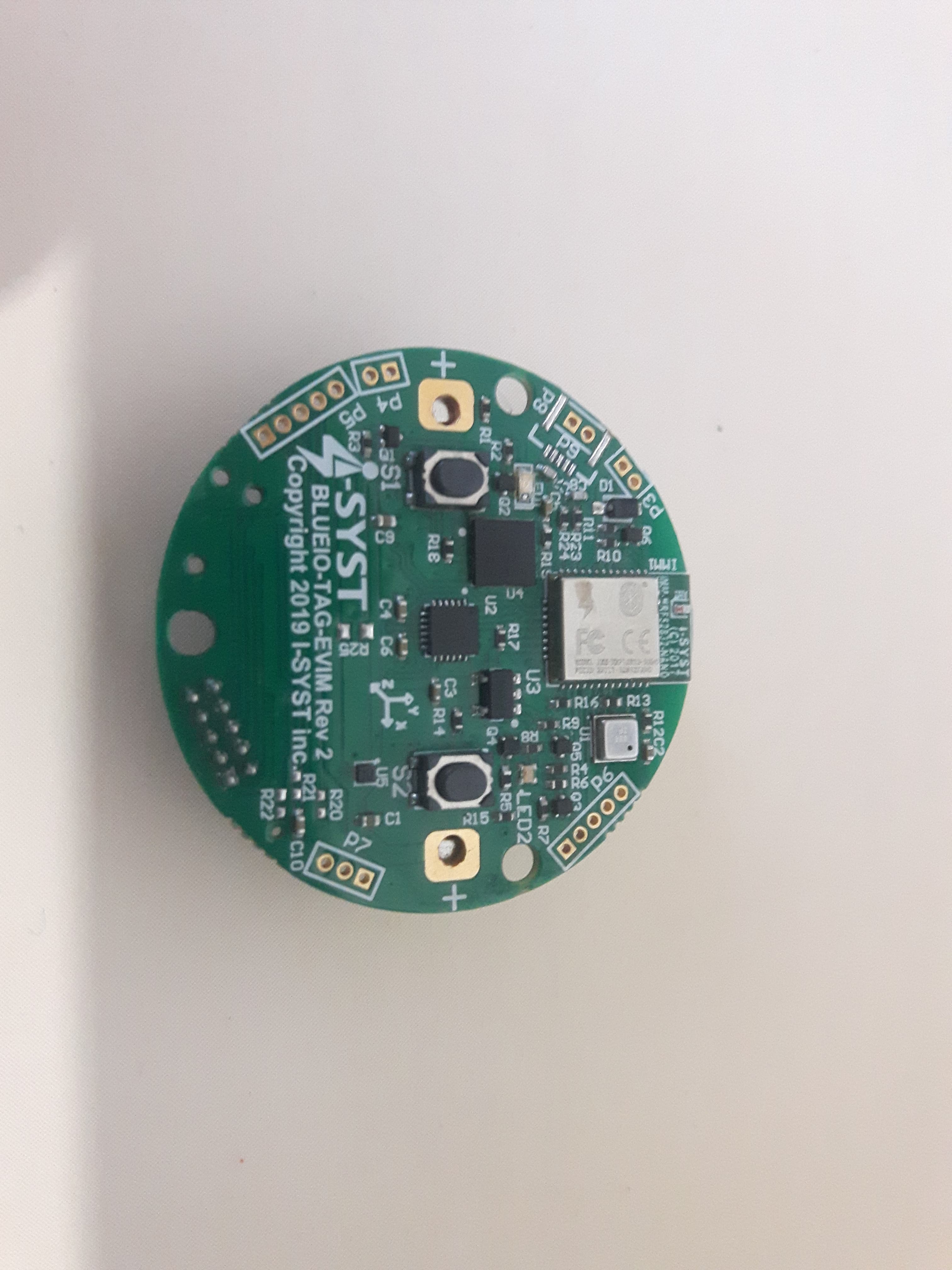
Now you are free to develop any android apps with BLUEIO-TAG-EVIM.
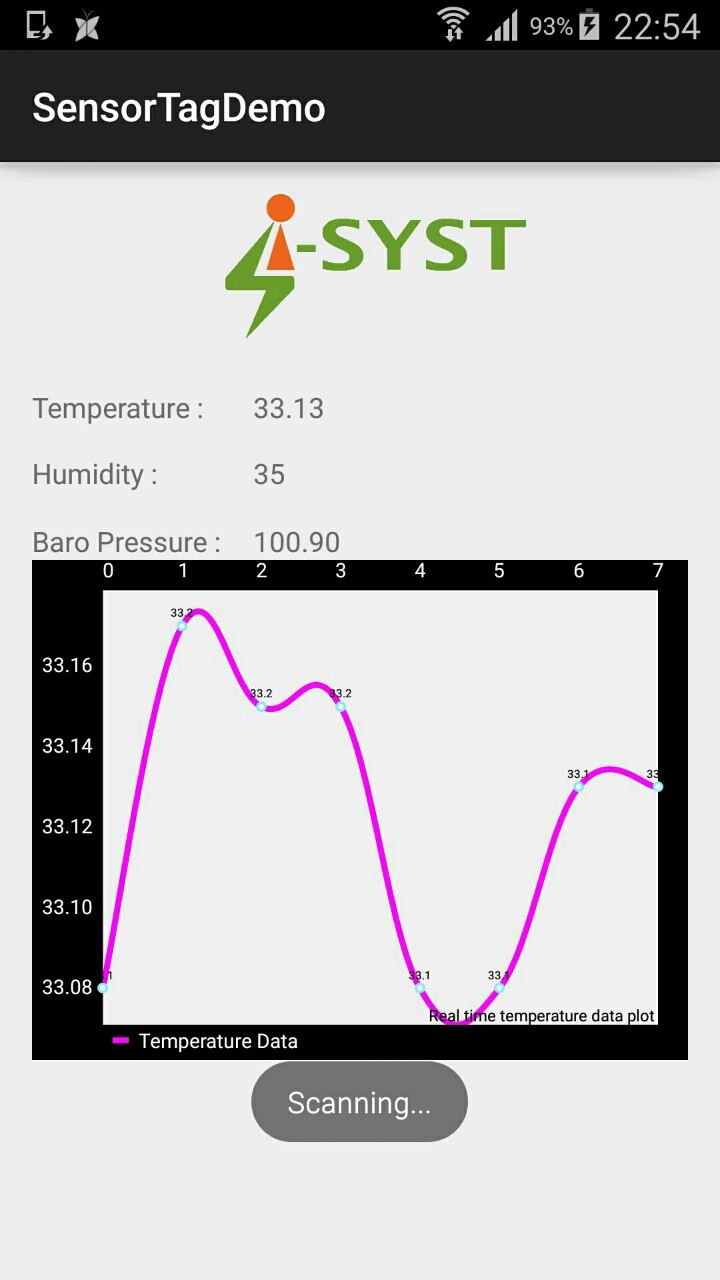
3. Building Android apps with BLE device
We now show some basic notes in Bluetooth low energy programming
This section is based on the book "Building Bluetooth Low Energy Systems" - Muhammad Usama bin Aftab
For more detail about building Bluetooth low energy applications, readers can find interesting things in this book: "Building Bluetooth Low Energy Systems"
1. Bluetooth permission
In the Android project structure, there is a file named AndroidManifest.xml which contains a high-level overview of the Android application. This file contains information about the activities, services, receivers, providers, libraries, and permissions. It is essential to give explicit permissions to Bluetooth. This can be achieved by providing the following line of code in the Manifest file:
<uses-permission android:name="android.permission.BLUETOOTH"/>
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/>
This permission ensures that the application is able to access Bluetooth. Another important permission for the proper execution of Bluetooth Low Energy scanning is:
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
2. Bluetooth Low Energy interfaces
There are three kinds of interfaces given for BLE in Android API 24. We will now introduce those interfaces in the this sections.
BluetoothAdapter.LeScanCallback
An interface required delivering the results of LE Scan. You need to implement the method onLeScan() which will return back scanned device, its RSSI (signal strength) and the scan record array. Later this object will be passed to the startLeScan method.
BluetoothAdapter.LeScanCallback leScanCallback = new BluetoothAdapter.LeScanCallback() {
@Override
public void onLeScan(BluetoothDevice device, int rssi, byte[] scanRecord) {
// Do something with the scanned data...
} };
startLeScan(leScanCallback);
BluetoothProfile.ServiceListener
This interface is used to notify the BluetoothProfile clients when they are connected or disconnected from the service. The must implemented methods for this interface are onServiceConnected() and onServiceDisconnected().
BluetoothProfile.ServiceListener leServiceListener = new BluetoothProfile.ServiceListener() {
@Override
public void onServiceConnected(int profile, BluetoothProfile proxy) {
//Do something upon receiving a service connection
}
@Override
public void onServiceDisconnected(int profile) {
//Do something upon receiving a service disconnection
} };
3. Bluetooth Low Energy classes
We will now discuss some important classes for Android BLE. These will help readers to understand I-Syst's Android examples.
BluetoothAdapter
This is the fundamental class which lets you accomplish basic Bluetooth tasks such as device discovery etc. You can get the adapter by calling:
// Initializes Bluetooth adapter.
final BluetoothManager bluetoothManager = (BluetoothManager) getSystemService(Context.BLUETOOTH_SERVICE);
mBluetoothAdapter = bluetoothManager.getAdapter();
This BluetoothAdapter is universal and the application can interact with it to transfer and receive data over the Bluetooth link.
BluetoothGatt
This class was added in Android API Level 18 and it enables the basic Bluetooth Smart communication. Functionalities like service discovery and reading/writing on characteristics are achieved using this class. In order to use this class, you first need to get the BluetoothGatt object by calling connectGatt() method on the scanned device.
BluetoothGatt mBluetoothGatt = device.connectGatt(this, true, mBluetoothGattCallback);
This mBluetoothGatt object can now be used to start the service discovery process and read/write the characteristics. In order to connect to the device, you need to describe a BluetoothGattCallback class which will ask you to override methods to do tasks when a certain event triggers.
BluetoothGattCallback
This is an important class in the Bluetooth communication which gives an interface to the developer to describe custom tasks performed upon a triggered event. For example, by implementing an onServicesDiscovered method, we can define the tasks to be performed after all the services are discovered. Similarly, onCharacteristicRead can be overridden to perform tasks upon reading a characteristic.
BluetoothGattCallback mBluetoothGattCallback = new BluetoothGattCallback()
{
@Override
public void onConnectionStateChange(BluetoothGatt gatt, int status, int newState) {
super.onConnectionStateChange(gatt, status, newState);
}
@Override
public void onServicesDiscovered(BluetoothGatt gatt, int status) {
super.onServicesDiscovered(gatt, status);
}
@Override
public void onCharacteristicRead(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic, int status) {
super.onCharacteristicRead(gatt, characteristic, status);
}
@Override
public void onCharacteristicWrite(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic, int status) {
super.onCharacteristicWrite(gatt, characteristic, status);
}
@Override
public void onCharacteristicChanged(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic) {
super.onCharacteristicChanged(gatt, characteristic);
}
@Override
public void onDescriptorRead(BluetoothGatt gatt, BluetoothGattDescriptor descriptor,
int status) {
super.onDescriptorRead(gatt, descriptor, status);
}
@Override
public void onDescriptorWrite(BluetoothGatt gatt, BluetoothGattDescriptor descriptor, int status) {
super.onDescriptorWrite(gatt, descriptor, status);
}
@Override
public void onReliableWriteCompleted(BluetoothGatt gatt, int status) {
super.onReliableWriteCompleted(gatt, status);
}
@Override
public void onReadRemoteRssi(BluetoothGatt gatt, int rssi, int status) {
super.onReadRemoteRssi(gatt, rssi, status);
}
@Override
public void onMtuChanged(BluetoothGatt gatt, int mtu, int status) {
super.onMtuChanged(gatt, mtu, status);
} };
BluetoothGattService
This class is responsible for holding different parameters and functionalities related to the Bluetooth Service. BluetoothGattService gives flexibility to the developer to get the characteristics related to that service which is significant for writing and reading data to and from the Bluetooth characteristics.
A service can be acquired by calling getService method on mBluetoothGatt object after connecting to a particular device:
BluetoothGattService service = mBluetoothGatt.getService(UUID.randomUUID());
An example of getting a random characteristic from a service object is:
BluetoothGattCharacteristic characteristic = service.getCharacteristic(UUID.randomUUID());
Note that the randomUUID() method is used for demonstration purpose and in the real-world application this UUID has to match the peripheral's UUID.
BluetoothGattCharacteristic
This class contains the functionality and parameters of a Bluetooth Characteristics. Methods related to reading and writing the data can be found in this class. Normally, a list of characteristics is acquired from the service object.
List gattCharacteristics = service.getCharacteristics();
// Loops through available Characteristics.
for (BluetoothGattCharacteristic gattCharacteristic : gattCharacteristics) {
// Do something with gattCharacteristic
}